Using Core ML in a Swift Playgrounds app
I love playing with machine learning, especially with the Core ML framework; I use it in Give Me a Sniglet to determine the validity of possible words, for example. On the Mac, Apple makes it easy to create or convert ML models for use in apps with Xcode.
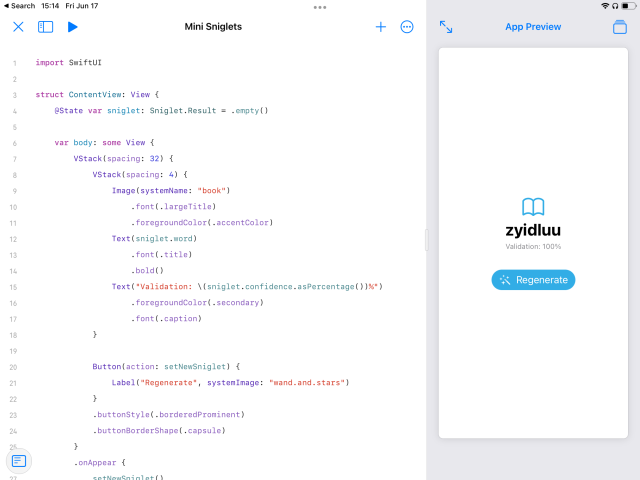
I love playing with machine learning, especially with the Core ML framework; I use it in Give Me a Sniglet to determine the validity of possible words, for example. On the Mac, Apple makes it easy to create or convert ML models for use in apps with Xcode, and the integration between these models with Xcode projects is phenomenal.
When playing around with the Swift Playgrounds app for the past week, I expected a similar experience for machine learning models because Playgrounds supported Core ML. To my surprise, this support is very limited; while Playgrounds can recognize an ML model file (.mlmodel
), it doesn’t do anything with the model. Thankfully, after some research and debugging, I’ve figured out how to use ML model files for machine learning in a Swift Playgrounds app project.
Unfortunately, there isn’t a way to create ML models on the iPad in the same way you can a Mac. However, you can create the model on a Mac and then transfer it to the app project file on iPad. It’s not the most ideal situation, especially for those that only have an iPad, but it is a working method. I hope Apple improves this in the future by bringing the Create ML app to iPad and adding the automatic class generation support to Swift Playgrounds.
To import a Core ML model into Playgrounds, I first created a dummy project in Xcode and imported the ML model file into the project. After a quick build (no running the target), I opened the ML model file in Xcode to view the generated class. I then copied the contents of the file into a new file in the Playgrounds app project. I also copied the ML model file over to the iPad using iCloud Drive (though any method will work, as long as it’s available in the file picker).
Next, in Playgrounds, I added the ML Model file as a resource and opened the Swift file I created that has the model’s generated class. I scrolled to the model’s class (which extends from MLModel
) and changed the class variable urlOfModelInThisBundle
to the following closure:
class var urlOfModelInThisBundle: URL {
let resPath = Bundle(for: self).url(forResource: "Model", withExtension: "mlmodel")!
return try! MLModel.compileModel(at: resPath)
}
ML model files aren’t compiled, and Core ML needs a compiled model to perform predictions. Unfortunately, importing a pre-compiled model into Playgrounds causes all sorts of issues, so the app needs to compile the model when launching for the first time. This code snippet will compile the ML model to a temporary directory that will be used for predictions later.
Once this initial setup is complete, I can use the Core ML model like I would in an Xcode project. As a test, I mocked up a miniature version of Give Me A Sniglet with the same ML model, and it works rather well.
I’d love to see this process improve the future. The current method is cumbersome and still requires a Mac to complete the job. I don’t know if Apple has plans for Swift Playgrounds 5, but improved Core ML support would be a bonus. Swift Playgrounds is a great way for anyone to learn iOS app development and experiment with new ideas in an easy to use fashion; machine learning should be a part of this goal.